Feature Overview
"KNUTIL" is a library for PICO-8 that contains functions that are frequently used in the games I have created.
I've kept the functions that I eventually needed in my production.
In this cart, I show you how the scene functions work with animations.
Z key: Execute the order command.
Up/Down: Select the order command.
SCENE MANAGER controls and replaces the order in which functions are called with a small number of tokens by using consecutive string instructions.
The generated SCENE can register a global function as an ORDER.
One of the registered ORDERS is retrieved by SCENE and the process is repeated for the specified length.
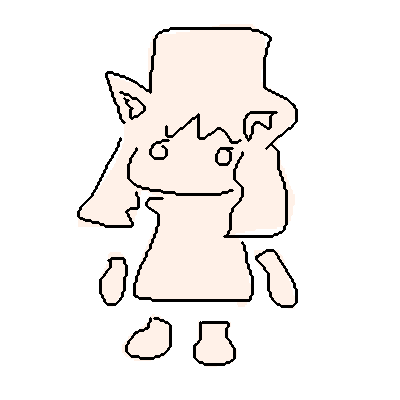
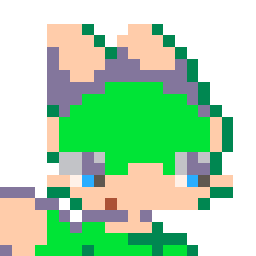
Hello, everyone.
A few days ago, a game I created called KONSAIRI was released on Steam!
http://store.steampowered.com/app/1448220/
There are still very few examples of PICO-8 games on the Steam platform as PICO-8 games.
I think that's because, as content, PICO8 titles are seen as lacking when compared to other game engines and those created in a free development environment with no restrictions.
But I challenge you to dream of being in the same store as a masterpiece game, despite its limitations.
I also wish to see more interest in PICO-8 in my part of Japan.
![]() |
[24x24] |

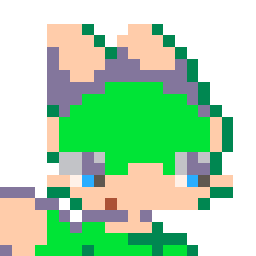
Hello.
Suggestion.
I would like the exported file to include the current config.txt (global_config.txt) as local_config.txt in the exported file.
This will allow us to play from the creator's ideal config in another user's environment.
"global_config.txt" refers to the config.txt that I've been using.
If both global_config.txt and local_config.txt exist, local_config.txt is assumed to take precedence.
Sequence of events
A question thread containing this
https://www.lexaloffle.com/bbs/?tid=39940
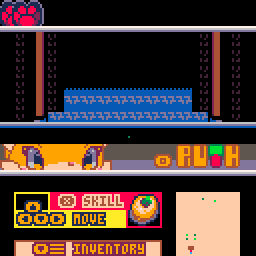
I'm developing KONSAIRI, a game I've been working on for a while now that I'm finalizing and checking throughout the game.
As I mentioned before, the goal of the game is to move back and forth between 16 different areas to reach the final destination.
However, it seemed to feel like the same old thing before you even reach halfway through the game.
That's why i added Dungeon mode, which changes the look and feel of the adventure dramatically.
Not only do you take damage when you touch an enemy, but there are hidden passages and hints scattered throughout the game that will help you get to the true ending.
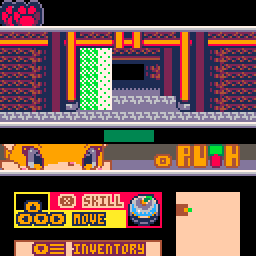
When you stand in front of an enemy, you will see a timing gauge.
It's not for attacking, but for getting through to the enemy's rear.
A well-timed "step move" will allow you to move forward with no damage.
If time passes, you will be damaged.
If you move away from the spot, you can avoid the danger.
Current Development
I'm building a relationship between NPCs and area map connections.
These are mainly for the latter 8 areas.
(The "keys" that NPCs have might be easier to understand.)
This game has a combined total of 16 areas.
There are also useful items that will help you move through such a large world.
These items can be found at the end of the first half of the game.
Then, the image below shows the map of the first half of the game.
Reduced map for 8 areas
Most of the maps are over 128*128 cell size.
The aspect ratio varies from area to area and the structure is challenging to explore.
Once you get your "KUWAI", use it! You can look around the entire area.
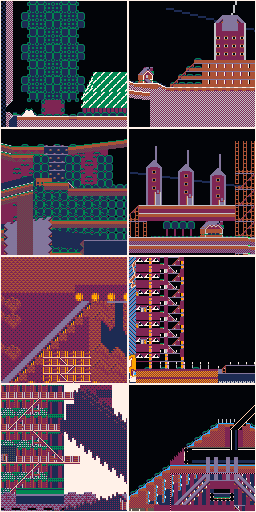
Actual map display
An animated tile is provided in each area.
These tiles bring about the effect of the wind.
You can use "PERSNIP" to change the wind (flow).
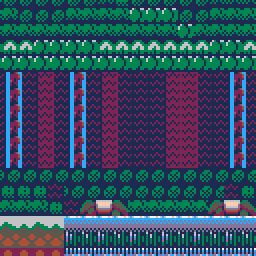
Awaking bells
Does the random value generation by srand() always produce the same pattern output as long as the same seed value is input?
Does the output pattern change due to a change in the environment?
For example, the upgrades, the amount of code, the timing of the exports, the machine or OS you're running...
So far I haven't been able to verify much and I haven't encountered the fact that the output results have changed, but I am concerned.
Thanks for your help.
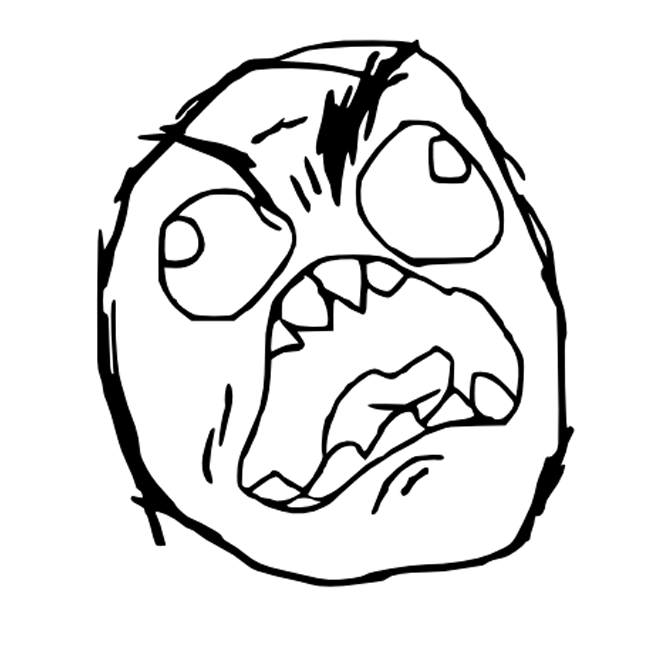
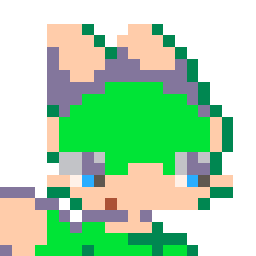
I'm working on a big project for PICO8.
Around the end of 2018, I learned about the scalability of multi-carting and continued to research and create it to this day.
We were able to give each of the four carts a role, rather than just being a data bank .
Cart 1: Title/Ending/3D dungeon scene
Cart 2: Platformer Scene
Cart 3: Cooking Scene
Cart 4: World Map Scene/Important Item Riddle Solving Operation
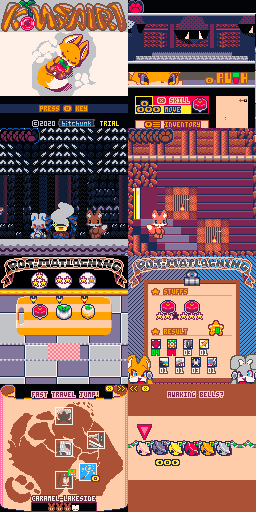
Because of these many factors, an hour or more of play is a must.
Data saves are automatic.
(Position when resume, growth and LIFE, possessions, time, status of NPCs helped, farmland, important items, veggies, history of veggies removed, history of enemies removed, and other things for cart-to-cart interaction).
Most of the game system is complete. I'm currently assembling the map for the later areas.
If anyone is planning an original, reasonably large game, PICO8 will be used as a prototype. It looks like they will progress the production and do the actual product in another game engine. I've seen some of those opinions. (Not my project.)
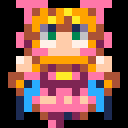
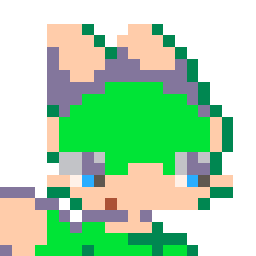
A new version has been released!
What the tool can do
- 3D modeling in 15x15x15 size.
- Save and load into a sprite sheet.
- Export the loading code from the sprite sheet.
- Export the drawing code of the 3D model.
Control are followings(WIP)
Reference
Examples of actual use
KONSAIRI
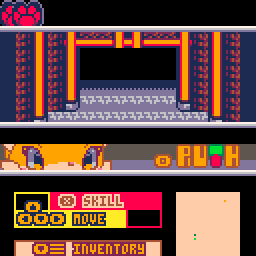
Export Star Model(Sample)
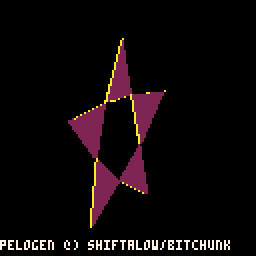
updated (v0.2.3)
- Added: Display of the next surface to be generated.
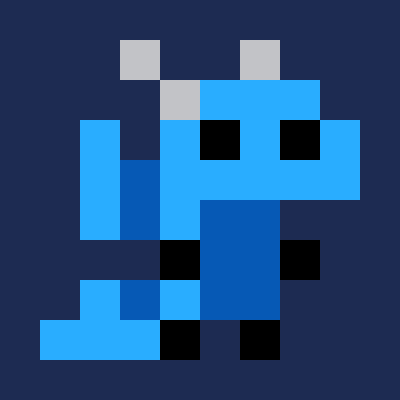
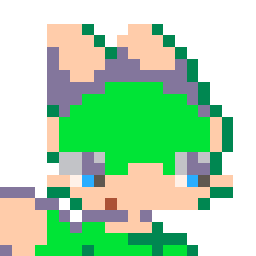
I am working on a small 3D modeling tool separate from the main game project.
The created 3D model is written to a sprite, and it is ready to be read.
However, operations and views are very confusing.
No such tool exists on the PICO-8 platform. (Or not seen)
I guess it's probably because there's an alternative and it's easier to produce.
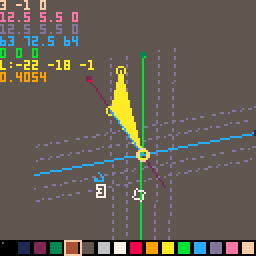
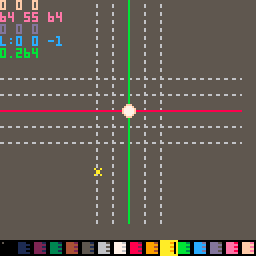
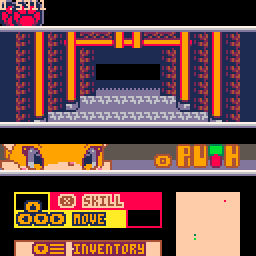
Would you like to take advantage of this tool, even if it is paid?


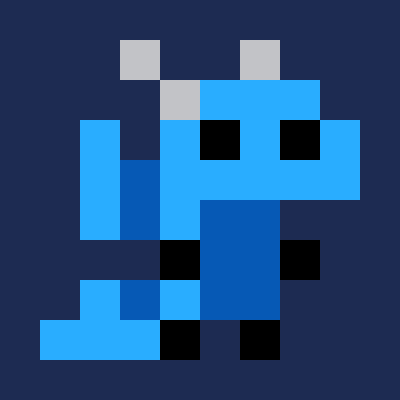
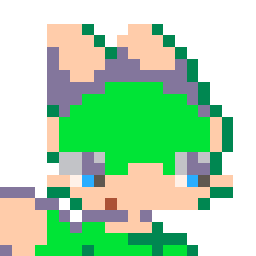
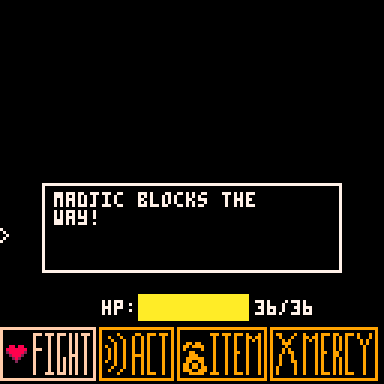
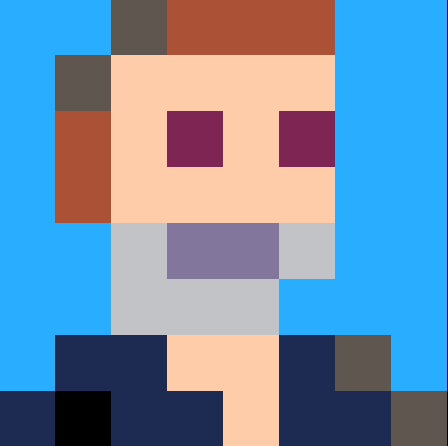
Feature Overview
This is a script that parses String data and converts it into a hash table.
- a single string can initialize many values.
- returns at least an empty table.
- elements can be added space-separated.
- spaces can be replaced with
\t
. - newline codes are ignored.
- newline can be replaced with
\r
. {}
specifies a table.key=val;
key{val}
specifies the key and value of the table.- if you enclose an empty string, the element will become nil.
- to initialize with an empty string, use
/0/
. - bool values, and hexadecimal strings are automatically normalized.
- the first layer can be initialized with global values by using
cat()
in_env
.
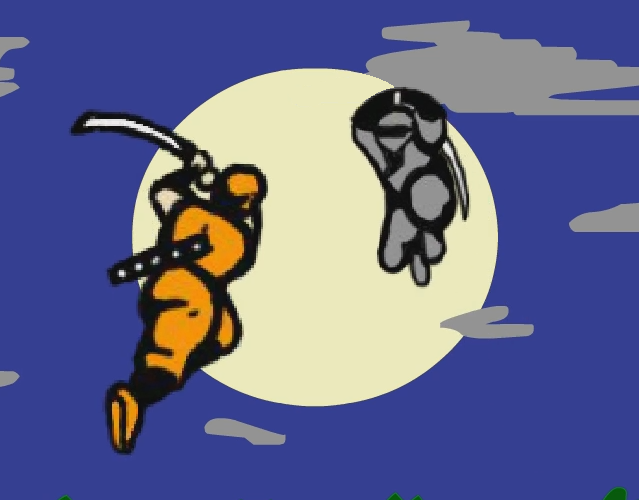

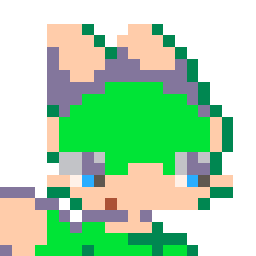
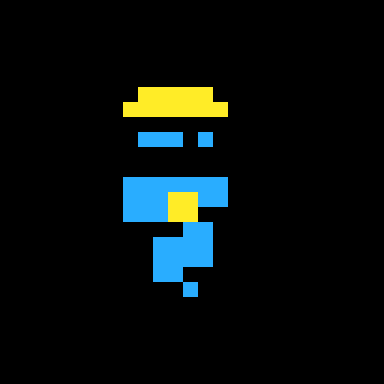
Feature Overview
- DMP() prints values, table internals.
- Stop the routine.
- Clear Screen.
- Display the contents of the specified table.
- The order in which the associative array table is displayed is undefined.
- Use the left, right, up, and down keys to scroll the screen and check the contents.
- This function consumes 168 Tokens.
local t={ 1 ,str="string" ,obj={"o","b","j","e","c","t"} ,{{{}}} ,{nil,true,false,function()end} } dmp(t) |
Symbol summary
# Number $ String % Boolean * Function(output only the "[Function]") ! nil(table pairs() skips nil, so it is not shown.) [ [size=16][color=#ffaabb] [ Continue Reading.. ] [/color][/size] ](/bbs/?pid=60679#p) |
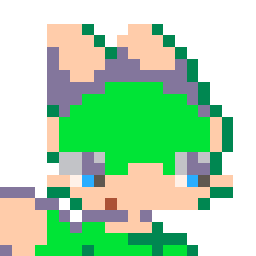
FillPatDraw FullColor version
This cart is tool that export code for drawing with FILLP() and RECTFILL().
The Exported data can draw that using without Sprite sheet.
You can also apply a check mask to an image by changing the value of _filppmask to a non-zero value.
Controls
[hidden]
Sprite sheet view
- Mouse Left Drag and Click
1 Select the rectangle to be the source of the delimited block.
2 Select the length of block from start to end.
3 Click Export Button for Confirm and Enter the export (p8l)file name.
In some cases there is a waiting time of 30 seconds or more.
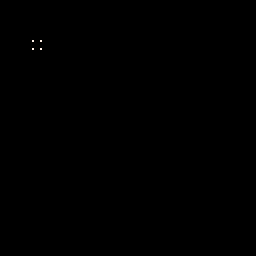
-
Right Click
Prev select mode. - Tab key
Open the Menu Window.
Menu
-
PLAY Button
Start Pattern Draw Preview -
SAVE Button
SAVE PNG file and Attach Cart data -
LOAD Button
LOAD PNG file and Attach Cart data - CLEAR!! Button
CLEAR Sprite sheet(not Attach Cart data)
Pattern Draw Preview
- Space key
Stop and Start the Animation. - Left Click
Step to Next BlockID. - Right Click
Prev to Back BlockID. - Tab key
Return The Menu Window.